Developing an application
Having installed ScriptX Server side printing and validated it is working correctly - where next in developing an application.
-
Review the implementation of the Validation Kit, this contains re-usable code for initialising the license and printing a document.
-
Review the main APIs that will be useful:
Summary of execution
A request will be received by the service - the service may be your own, or may be IIS (or similar web server). The service will route the request to a handler - for example in an MVC application that may be a method on a controller that will return an ActionResult, in a WebForms application it may be a Page_Load handler on an ASPX page or in a an ASP.NET application it may be a WebAPI method.
However the call is routed, your hander code will execute the following:
- Instantiate an instance of Security Manager.
- Call the Apply method to enable the licensed features of ScriptX.
- Instantiate the MeadCo ScriptX Factory object.
- Obtain the printing object.
- Configure the required parameters, for example the printer to use, the paper size, the bin, headers, footers etc.
- Determine the document to be printed, or dynamically construct it in some way.
- Call the PrintHtml method to queue printing of the document.
- Shutdown the printing object to ensure release of resources.
At this point your handler should return appropriate content to the user. ScriptX will process the printing in the background on the server. As other requests are received they will be added to the queue and processed in order.
NOTE: Do not use the WaitForSpoolingComplete method after the call to PrintHTML. This method is intended for use on client side printing when you need to control the sequence of events before, say, closing a window. In a server scenario it will cause the current thread to block.
Developing an application with Visual Studio (C#)
A simple application will have a single action/page on the server that performs printing for the application. To illustrate use, we will omit a lot of error checking and also will omit using utility classes that would hide some of the complexity in error checking.
Reference the components
Use Add Rerference ... for the project to reference the COM Components MeadCo Security Manager and MeadCo ScriptX:
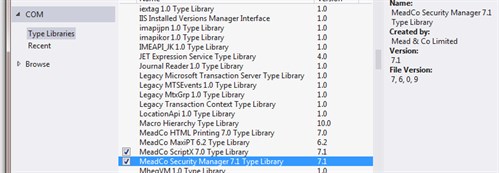
Apply the license
The license must be pre-installed on the machine and must then be referenced and applied to the service/application to enable the advanced printing features:
@{
Layout = "~/_SiteLayout.cshtml";
Page.Title = "Document printed";
// apply the scriptx license
var secMgr = new SecMgr.SecMgr();
secMgr.Apply("", ConfigurationManager.AppSettings["licenseGUID"],
int.Parse(ConfigurationManager.AppSettings["licenseRevision"]));
...
}
Obtain the ScriptX printer object
@{
...
var factory = new ScriptX.Factory();
var printer = (ScriptX.printing) factory.printing;
...
}
Configure the printer
@{
...
// Apply the required settings, this can include the printer,
// papersize, copies, orientation, bin etc.
printer.header = "ScriptX Printing - Printing at the Server";
printer.footer = "Printed at the server";
...
}
Construct a document
For simplicity a url within the application will be used. Note that the absolute uri must be obtained; a relative reference cannot be used since the print component knows nothing about the current context.
@{
...
var path = VirtualPathUtility.ToAbsolute("~/About");
var uri = new Uri(HttpContext.Current.Request.Url, path);
var url = uri.AbsoluteUri;
...
}
Print the document
The print job is queued with a call to PrintHtml - do not attempt prompted printing as there is no one there to click print!
@{
...
printer.PrintHTML(url, 0);
...
}
After queuing the print, the ASP.NET page will continue processing and return to the user. On the server, the requested docoument will be downloaded and printed.
Dispose the components
The component references must be unwound so that the component is released and the component server process will shutdown.
@{
...
printer = null;
factory.Shutdown();
...
}
The above is the minimum requirement. The component print server proces will shutdown after the server process performs garbage collections and a period of inactivity or when the calling server process (e.g. IIS) is shutdown.
Please see the Validation Kit for example code where the server is shutdown more quickly.
Putting it all together ...
@{
// apply the scriptx license
var secMgr = new SecMgr.SecMgr();
secMgr.Apply("", ConfigurationManager.AppSettings["licenseGUID"],
int.Parse(ConfigurationManager.AppSettings["licenseRevision"]));
var factory = new ScriptX.Factory();
var printer = (ScriptX.printing) factory.printing;
// Apply the required settings, this can include the printer,
// papersize, copies, orientation, bin etc.
printer.header = "ScriptX Printing - Printing at the Server";
printer.footer = "Printed at the server";
var path = VirtualPathUtility.ToAbsolute("~/About");
var uri = new Uri(HttpContext.Current.Request.Url, path);
var url = uri.AbsoluteUri;
printer.PrintHTML(url, 0);
printer = null;
factory.Shutdown();
}
::> Guide for Printing in Applications